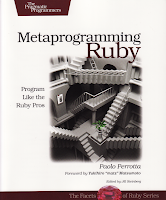
Friday, 22 October 2010
Metaprogramming Ruby
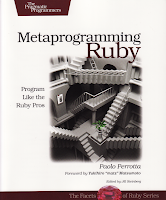
Sunday, 17 October 2010
TeX code generation with perl
#!/usr/bin/env perl
sub initialize{
$text = '\documentclass{article}'."\n";
$text .= '% Created by '."\n";
$text .= '\begin{document}'."\n";
}
sub usepackage{
$text = '\usepackage{'.$_[0]."}\n";
}
sub finalize{
$text = '\end{document}'."\n";
}
sub itemize{
$text = '\begin{itemize}'."\n";
$text .= "\t\item"."\n";
$text .= '\end{itemize}'."\n";
}
sub enumerate{
$text = '\begin{enumerate}'."\n";
$text .= "\t\item"."\n";
$text .= '\end{enumerate}'."\n";
}
sub figure{
$text = '\begin{figure}'."\n";
$text .= "\t".'\includegraphics{}'."\n";
$text .= '\end{figure}'."\n";
}
sub table{
$text = '\begin{tabular}'."\n";
#$text = 'table';
$text .= '\end{tabular}'."\n";
}
sub tabbing{
$a = '\begin{tabbing}'."\n";
$a .= '\end{tabbing}'."\n";
}
sub section{
$a = '\section{'.$_[0]."}\n";
}
sub subsection{
$a = '\subsection{'.$_[0]."}\n";
}
sub equation{
$a = '\begin{equation}'."\n";
$a .= $_[0];
$a .= '\end{equation}'."\n";
}
And a simple example is given below:
print &initialize;
print &usepackage("graphicx");
print &itemize;
print &enumerate;
print &figure;
print &table;
print &finalize;
Friday, 8 October 2010
Book Review: Foundations of GTK+ Development
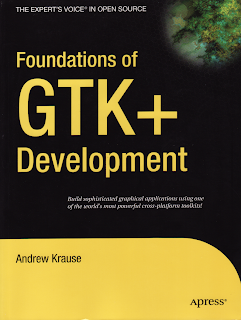
Wednesday, 6 October 2010
xcf2pdf
#!/usr/bin/python
from subprocess import *
import re
def convert(file):
p = Popen(['convert', file, file[:-3]+'pdf'], stdout=PIPE, stderr=PIPE)
p = Popen('ls', stdout=PIPE, stderr=PIPE)
ls, error = p.communicate()
files = re.split('\n', ls)
for i in files:
if i[-3:]=='xcf':
convert(i)
There are a few features missing and known bugs, e.g. it will convert every layer of the xcf file to a different page in the same pdf. (Beware of the python code formatting)
GGGears
In many engineering applications engineers have to calculate how gears are working under stress. GGGears is a free software that does exactly this by means of the finite element package GETFEM++ and the mesh generation package GMSH, both also free software. It supports both 2D and 3D mesh generation and solver. The user does not need to know anything about finite elements or mesh generation; he/she just need to insert the geometrical data of the gear systmem.
The installation of the software can be easily done in Ubuntu by first adding the repository